Blockchain Investment Guide: Tips to Invest in Blockchain Technology
By
Guest Author
If you have not been living under a rock for the past eleven years, then you have probably heard of blockchain. With thousands of new and existing cryptocurrency tokens available in the market, it is just starting its way into completely transforming the world around us. Even though it started its journey to provide an ... Read more
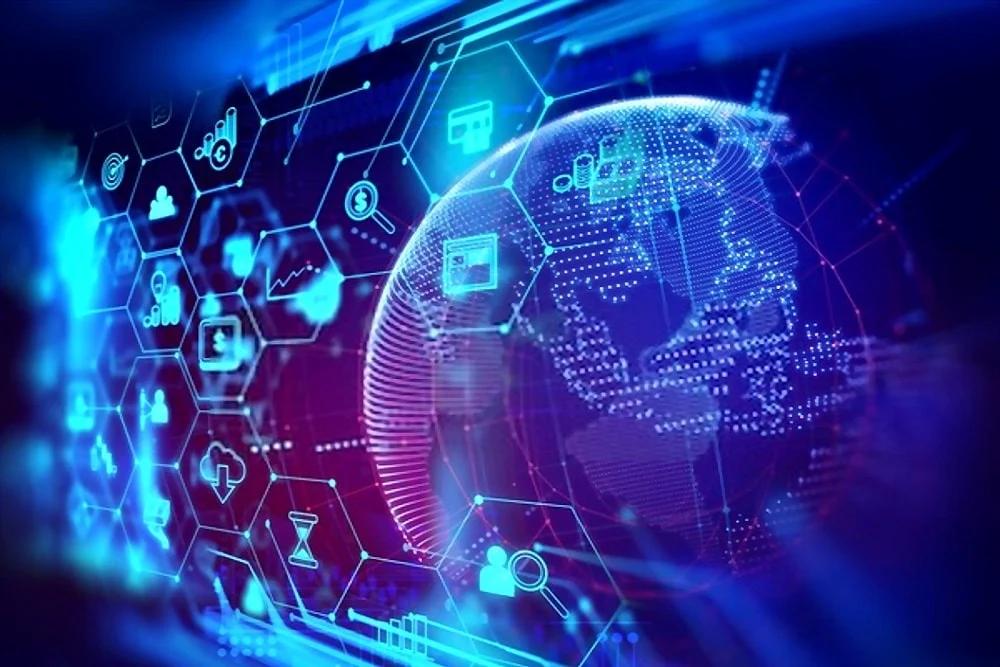
If you have not been living under a rock for the past eleven years, then you have probably heard of blockchain. With thousands of new and existing cryptocurrency tokens available in the market, it is just starting its way into completely transforming the world around us. Even though it started its journey to provide an alternative to the regular currencies via cryptocurrencies just 11 years ago, it is already making its mark in almost every industry possible.
Global blockchain technology revenues are predicted to climb to over 23.3 billion U.S. dollars in size by 2023. So you are tempted enough to invest in this new and radical technology and you certainly should! However, any investment can be troublesome if you do not have adequate knowledge of the sector you are investing in. We are here to help you understand the basics of the technology itself, along with how to invest in it so that you can make an informed decision.
What is blockchain?
In very simple terms, “blockchain” is a series of time-stamped “blocks” which contain digital information, and are stored in a public database using cryptographic principles or “chain”. There are 3 types of digital information stored in each of these blocks – information about a transaction, information about the participant of that transaction, and special information (hash) stamped to each block so that they distinguish from one another.
Blockchain technology is so special because of the following incredible qualities it possesses –
- Unlike the usual storage over the internet, instead of one, the record of data is stored in a cluster of computers. No central authority like the Government or bank is controlling them – hence, this data is decentralized.
- A “block,” before being added to the “chain” is verified by potentially millions of computers distributed around the network, which makes this verification thorough and more accurate.
- Once data is stored in a blockchain, it cannot be altered in any way. No amount of authority or secret hacking can change this fact. Therefore, transactions over a blockchain are absolutely secure.
- The information stored in the blockchain is shared publicly, so everyone can see the information there. However, the user data is hidden under obfuscation called “digital signatures” unique to each user. Thus blockchain information is public, at the same time is private.
- Since it is decentralized, it carries no heavy transaction cost, making it cheaper than traditional transactions.
What are the main areas of blockchain investment?
Whenever someone talks about blockchain applications, your mind has a tendency of going directly to cryptocurrencies like Bitcoin. However, blockchain is the only technology behind cryptocurrencies, not cryptocurrencies themselves. When considering blockchain investment, you can invest in a number of sectors –
- Cryptocurrencies: You can simply buy cryptocurrencies like Bitcoin (BTC), Ethereum (ETH), or Ripple (XRP) at a low cost and sell at the time when their value rises. This is the most common method of investment in blockchain technology.
- Stocks: You can use investment funds like exchange-traded funds (ETFs) such as Reality Shares Nasdaq NexGen Economy ETF (BLCN), Innovation Shares NextGen Protocol ETF (KOIN), Amplify Transformational Data Sharing ETF (BLOK), etc. which lets you diversify your investment by spreading money across multiple stocks as well as allows you real-time trading. You can also invest in individual stocks like Overstock (OSTK), Nvidia (NVDA), Mastercard (MA) if you want to go big on your own.
- Blockchain Startups: This may not be the most convenient method of investment, but it might just be the most profitable one if you play your cards right. Because of the amazing features blockchain technology holds, it can revolutionize and reinvent quite a few sectors like banking, healthcare, elections, air travel, sports, cybersecurity, human resources, sales, real estate, music and other entertainment areas, ride-sharing, energy conservation, and many more. Blockchain startups usually raise money via Initial Coin Offerings (ICOs) but the latest method is called Initial Exchange Offerings (IEOs). Over the past few years, there have been several blockchain consulting start-ups like Guardtime, Chronobank, eXo, Openbazaar, Propy, Opus, Arcade City, Acciona Energy, etc. which took the world by surprise and have been transforming the world with their innovative ideas.
Risks in blockchain investment
No matter how amazing blockchain investment sounds, there are a few drawbacks linked to it. The cryptocurrency market is volatile and prices fluctuate regularly. The crypto market has lost $340 billion of value since the start of 2018. On the other hand, investors are pulling off of all blockchain applications as well – so far, traditional venture capital investments in blockchain companies have totaled $784 million via 227 deals, according to CB Insights, which will have almost a 60% drop in profits for businesses invested in this technology. There is no doubt blockchain does attract some amount of risks.
Frauds
- False whitepapers: In order to raise crypto capital for a start-up, all someone needs to do is draw up a detailed white paper document and convince people to invest via ICO or IEO; a structured company is not required. If you do not do your research, you may lose a fortune. However, since there is no authority you can complain to, the money you lose is gone forever.
- Hacking: Hacking poses a similar threat. The cryptocurrency industry lost an estimated $11 billion via exchange and custodian hack since the last decade, and that money is gone for good.
Market manipulation
Market manipulation, which is absolutely illegal in the real stock market, poses no problem in the virtual currency world. Investors, very unethically, quite often inflate the value of the currency only to sell it off at its peak. As crypto has a pretty small market cap, this scam is easy to pull off.
Risky investment
- You might lose assets: Not every startup ends up becoming Amazon or Netflix. Even if the startup you invest in is legit, there is no guarantee it will become successful; in fact, a lot of them shut down quickly enough for you to earn anything at all. ICOs are often less successful than other businesses.
- It is a long-term investment: Once you invest in any blockchain consulting startup or project, you are in it for the long haul. They usually take some time to even start working, and even longer to be really successful – however big ROIs they end upbringing in the future.
- Highly volatile: As for cryptocurrencies, there is no governing body to expand or limit the money supply to meet changing events, and also no mechanism to prevent widespread price manipulation. This makes crypto highly volatile, i.e, the value of them rises and falls in a very disorganized manner. Bitcoin shot up in value from $1,000 per Bitcoin on January 1, 2017, to $19,783.06 in mid-December of that year, and then again the value went down by 80% in between January and September in 2018.
- Cryptocurrency paradox: Being decentralized, the price of a cryptocurrency is only determined by supply and demand and supply differs widely between exchanges. If someone buys a lot of one form of currency on a smaller exchange, investors on other exchanges will notice and buy the same currency, triggering market-wide inflation. This creates the “Cryptocurrency paradox.” People investing in crypto buy and hold them because they want their value to go up, but unless they use their crypto the value will remain the same. On the other hand, unless the crypto is used, its value will keep falling, and investors will start selling to avoid further losses. This goes on and on, leading to the inevitable “death cycle.”
Top tips to invest in blockchain applications
Knowing the risks you might have to face once you get into blockchain investments is not a reason to pull yourself back. You can use the knowledge to your advantage. If you are careful and patient, you surely will see profits – you do know about people being millionaires by investing in the technology and surely that is one of your biggest motivations for getting into it in the first place. Let us see what you can do to minimize your risks and get to profits:
- Do your own research (DYOR)
There was a reason why you are told to do your homework in school so that you understand what is going on in the next class. Investments are not really that different from those classes, and doing that homework can actually save you hundreds of dollars and might end up earning you a million more. Copying someone else’s work here can get you in big trouble. Never, ever invest in any form of blockchain because someone else suggested a certain company or simply because of peer pressure. The path less traveled can indeed be the winning one, and so the hard work needs to be your own – sorry folks, there is no quick fix in this. Make sure you research whatever you are investing in.
- Read the white paper yourself, and make sure you understand it well
You should get it registered well into your mind first that you are investing in something that has the potential to fail; so you need to know for sure if it is going to earn you any ROI ever. The white paper should contain every minute detail of the project written out for the investors. If the white paper is poorly written, there is a possibility that the team itself does not comprehend their own project and how it is going to bring profits; there are a lot of groups like this out there. Even though they may not be frauds, their projects may not be worth investing in.
- Figure the actual value the project will bring you
If the project is bringing any utility to the ecosystem, only then it is even worth considering. For example, Ethereum, even though launched soon after Bitcoin, took off quickly because apart from being traded as a digital currency, developers could run their own distributed applications (dapp) on the platform itself. So it did not necessarily compete with bitcoin, but made the technology of blockchain available to everybody, preventing its limitation to financials only.
Also have a lookout for projects which are trying to solve the main issues blockchain technology promises to solve – privacy, scalability, and interoperability.
- Analyze the project to learn if it actually needs ICOs
Not all start-ups actually need blockchain or crypto tokens – a project can do an ICO simply to raise money with no guarantee of giving you anything substantial in return. So how do you know if a project really needs blockchain or token? For this, you will need to understand the role of a token in a project as well as your rights as an investor:
- Right: As an investor of a token, you gain certain rights in that ecosystem. For example, if you have DAO coins, you could have voting rights inside the DAO to decide the funding for a particular project.
- Value exchange: Tokens create their own internal ecosystem – it is one of the most important roles of them. There can be buyers and sellers within the confines of the same ecosystem trading value. This way, you can earn rewards when you complete a task.
- Internal privileges: You can gain rights to certain functionalities of a system by possessing a particular amount of tokens in an ecosystem. For example, you can enjoy the benefits of Golem supercomputers by holding a certain number of Golem tokens (GNT).
- Functionalities: The tokens can enable you to enrich the user experience within the confines of your ecosystem. For example, Brave investors can use their tokens (BAT) to enrich the customer experience by adding advertisements or other services on the Brave platform.
- Currency: The tokens can be used as store-of-value to conduct transactions inside as well as outside their ecosystem.
- Earnings: The profits and other related financial benefits of a particular project should be equitable among investors.
Now, for the tokens you are investing in to have maximum utility, they should check off most or if possible, all of these boxes. If your tokens have low utility, they have more velocity which means people will sell them off too quickly for the network to achieve a productive enough average value and you do not want that. Read market research reports, market analyses, articles in crypto news sites, as well as comments written by analysts and experts to decide on your pick.
- Watch out for the obvious sign of scammers
It is a given that your investment in blockchain is risky, with no exceptions. So you will need to think and plan what will gain you the most profits in the long term – if you are thinking about getting rich real quick, you might fall victim to scammers. Invest in coins whose team shows you a transparent technical vision, an active development team, and an excited community. Watch out for the obvious signs of a scammer:
- Investigate the team
Google is at your disposal for a reason, use it. If the team is genuine, the members usually have LinkedIn profiles. Check if they are experienced in the technology if they have been involved in any successful ICOs before if they have been recruited in reputed companies before, if they have good recommendations, and all the necessary details you would research if you were to hire an employee. Also, do a Google image search for the members to avoid being “catfished” and confirm their authenticity. After all, if you are an investor, you are technically the boss, so do not shy away from interviewing the team well. Moreover, the internet is a fishy place and you really are not going to meet any team member face-to-face; so this is your right.
- Beware of the Pyramid Scheme
A pyramid scheme is where you are promised money in exchange for recruiting more people into the business. As more people are recruited however, the process of it becomes invalid as too many members substantially reduce the profit – hence the business falls down. If an ICO promises you “guaranteed returns”, it is most definitely a scam; you well know by now that there are no guarantees in the blockchain world. A very infamous example of such was – Bitconnect. Projects like Bitconnect left a lot of investors in debt, so beware.
- Github repository
A Github repository is where you can see the progress of the project. Before investing, check out if the developers are seriously working on the project or not.
- Invest only what you are mentally prepared to lose
Let’s face it, investing in blockchain applications, whether it is in start-ups or in crypto, is a lot like gambling. So if risk-taking is not your thing, do not invest in blockchain or you might end up in the psychologist’s office for anxiety issues. As we already mentioned earlier, cryptocurrencies are volatile and the market goes through frequent ups and downs. So do not put in your life’s savings into it – there are many not-so-volatile options for that.
Also, there is no hard and fast rule that you will have to invest a certain lump sum. Before investing, prepare yourself with the possibility that your money might completely go down the drain. Make a budget accordingly and put in only what you are okay with losing, even if that is just $1o.
- Back up, then back up your backups
Diversification is the key. Do not put all your eggs in one basket, especially when it comes to investment. The best investors in the world always have kept more than one door open for income. There are numerous options for you to choose from whether you want to invest in blockchain-based start-ups, stocks, or cryptocurrencies. Spread your investment over more than one currency or ICO. Even if the market takes a dip, there is a good chance that not all of them together will fall face down.
- Consider security token offerings (STOs)
To put simply, security tokens offerings (STO) are cryptographic tokens that pay dividends, share profits, pay interest, or invest in other tokens or assets to generate profits for the token holders. Instead of cryptocurrencies, you may consider investing in security tokens. This way, you will not have to worry about any legal consequences, they have their own built-in exchange systems and active communities, they provide you with instant liquidity on a regulated exchange, and you get direct access to a broad investor pool. Overall, the risks of investment will be lower if you get into them.
Learn the difference between STO, ICO, and IEO
- You may buy cryptocurrencies without actually buying them
If you want to invest in blockchain but do not want to deal with the volatility, there is another option available for you – you can bet on a cryptocurrency’s price without actually buying a cryptocurrency with the help of investment vehicles like XBT Tracker, Bitcoin ETI, etc. This is another way for you to stay within the reach of the legal system – if something goes wrong, you have someone to point blame at.
- Consult an expert; you may not be one in this case
All being said, it should be evident by now that blockchain is complicated technology and the methods of investing in it are complex. While that is no reason for you to stay away from investing in them, it is always a smart idea to bring in some experts for advice. You might also face taxation issues once you start earning from this, and taxation in crypto is completely different from the regular taxing system. So listening to professionals might just get you ahead of the others in the race.
Conclusion
Slow but steady wins the race – all investments are the same that way. Follow the guidelines above and more importantly, follow your instincts instead of your heart, take risks in a calculated manner – and there is a very good chance that you will see success in blockchain investment.
Bhawna is a Community Manager at Blockchain Australia by profession and storyteller by nature. A reader at night and analyst during the day, her areas of focus are marketing, tech, and startups. You can follow Bhawna on Twitter, LinkedIn, and Medium for her invaluable marketing tips and recommendations.

Follow us on Google News
Get the latest crypto insights and updates.
Related Posts
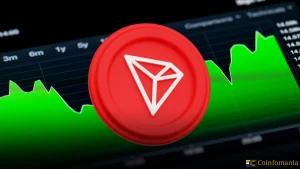
TRON Hits Record $1.93T in USDT Transfers During Q2 2025
Shweta Chakrawarty
Technical Writer
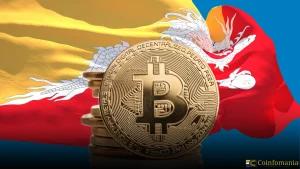
Bhutan Moves $12M in BTC to Binance, Extends 513 BTC Sell Spree
Shweta Chakrawarty
Technical Writer
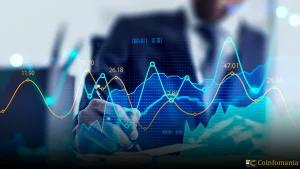
Trader @qwatio’s $334M Short Wipe Pushes Total Losses to $25.8M
Shweta Chakrawarty
Technical Writer